Sorry if my code is hard to read. I haven't programmed much since I took c++ last year! I'm trying to write a program that summarizes results for 2x round robin parlays. Input the number of teams, the price for each team, and your unit size, and output each individual bet. The code for this looks like:
Easy enough. Now I would like to add an output that summarizes the results if a certain team loses. The algorithm I have written is:int main()
{
int num;
cout << "Enter the number of teams:\n";
cin >> num;
string team[num];
double price[num];
int unit;
double mult[num];
double bet[50][50];//arbitrarily set to 50x50. Each bet will have a value based on the unit size and the multipliers corresponding to that bet.
double wins = 0.0;
double losses = 0.0;
double total = 0.0;
cout << "Enter the teams and the price one at a time.\n";
for (int i=0; i<(num); i++)
{
cout << "Team: \n";
cin >> team[i];
cout << "Price: \n";
cin >> price[i];
**
cout << "Enter unit size:\n";
cin >> unit;
for (int i=0; i<(num); i++)
{
mult[i] = 1 + (100/(price[i]));
**
for (int j=0; j<(num-1); j++)//creating each combination of 2 team parlay based on corresponding multipliers
{
for (int i=(j+1); i
{
bet[j][i] = unit * ((mult[j]*mult[i])-1);
**
**
cout << "Summary: \n";
for (int j=0; j<(num-1); j++)//outputting every combination and how much is to be won for each
{
for (int i=(j+1); i
{
cout << "\n" << team[j] << "\n" << team[i] << "\n Bet " << unit << " to win " << bet[j][i] << ".\n";
**
**
return 0;
But for some reason, the program compiles but stops running when it reaches the first loop. Which is odd because I traced the loop and still don't see how it's not working. Anyone know why, or know of a better algorithm to use? Thanks!for (int k=0; k<(num-1); k++)
{
for (int j=k; j<(num-1); j++)
{
for(int i=0; i
{
if ((j=k) or (i=k))
losses = unit * (num-1);
if (((j>k) or (jk) or (i
wins = wins + bet[j][i];
**
**
total = losses - wins;
cout << "If " << team[k] << " loses, you lose " << total << ".\n";
total = 0.0;
wins = 0.0;
**
wins = 0.0;
total = 0.0;
for (int j=0; j<(num-1); j++)//summarizing results if each team wins
{
for (int i=(j+1); i
{
wins = wins + bet[j][i];
**
**
cout << "If all teams win, you win " << wins << ".\n";
EDIT: This came out all whack, fixing... The "**" should just be brackets. Don't know why sbr is f*cking up the code so badly.
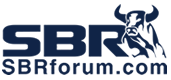